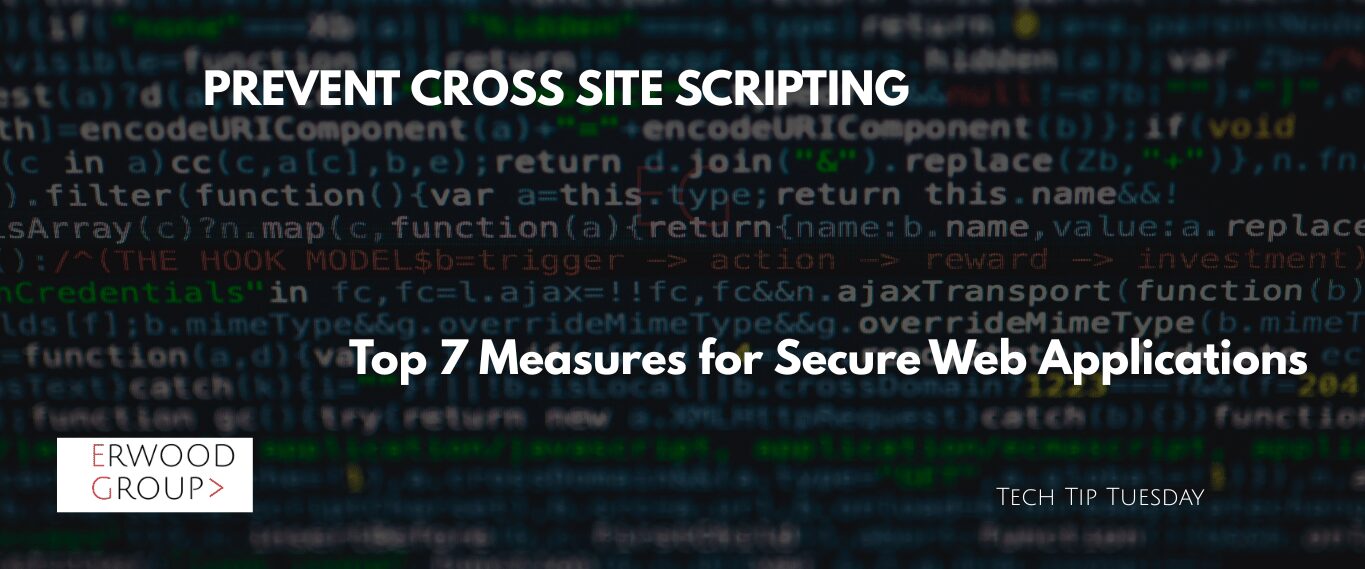
Top 7 Measures for Secure Web Applications
Cross-site scripting (XSS) attacks are among the most common web vulnerabilities, allowing attackers to inject malicious scripts into web pages viewed by unsuspecting users. These scripts can steal sensitive data like passwords, hijack user sessions, deface websites, or spread malware. For developers, especially beginners, preventing XSS is critical to building secure applications that protect users and maintain trust. This article, How to Prevent Cross-Site Scripting (XSS) Attacks: Top 7 Measures for Secure Web Applications outlines seven essential measures to safeguard your web application against XSS attacks. Each measure includes step-by-step instructions and beginner-friendly code examples to help you implement robust defenses, ensuring your application remains secure.
How to Prevent Cross-Site Scripting (XSS) Attacks: Top 7 Measures for Secure Web Applications
- Input Validation and Sanitization
Input validation ensures that data entering your application meets strict criteria, while sanitization removes or neutralizes potentially harmful content. Together, they prevent malicious scripts from being processed.
Step-by-Step Instructions:
- Identify Input Sources: List all places users can submit data, such as forms, URL query strings (e.g., ?search=query), or file uploads.
- Define Rules: Specify what each input should look like. For example, an email field should match a pattern like name@domain.com, and a username should only contain letters, numbers, or underscores.
- Validate on the Server: Use server-side code to check inputs against your rules. Client-side checks (e.g., in JavaScript) can be bypassed, so server validation is essential.
- Sanitize Inputs: Remove or escape dangerous characters like <, >, or “. Use libraries to avoid errors.
- Test Your Rules: Submit invalid or malicious inputs (e.g., <script>alert(‘hack’)</script>) to ensure they’re rejected or cleaned.
Code Example (PHP for Beginners):
<?php
// Step 1: Get user input (e.g., from a form)
$username = $_POST['username'];
// Step 2: Validate - allow only letters, numbers, underscores (up to 20 characters)
if (!preg_match('/^[a-zA-Z0-9_]{1,20}$/', $username)) {
die('Invalid username. Use letters, numbers, or underscores only.');
}
// Step 3: Sanitize - remove any HTML tags or scripts
$clean_username = htmlspecialchars($username, ENT_QUOTES, 'UTF-8');
// Step 4: Use the clean input (e.g., store in database)
echo "Welcome, $clean_username!";
?>
Explanation for Beginners: The preg_match function checks if the username follows our rule (letters, numbers, underscores). If not, the script stops with an error. htmlspecialchars converts characters like < into <, making them safe to display. Always do this on the server (PHP runs on your server, not the user’s browser).
- Output Encoding
Output encoding transforms user data into a safe format before displaying it, preventing the browser from executing it as code.
Step-by-Step Instructions:
- Find Output Points: Identify where user data appears in your app, like in HTML, JavaScript, or CSS.
- Choose the Right Encoding: Use HTML encoding for HTML content, JavaScript escaping for scripts, and URL encoding for links.
- Encode at Output Time: Apply encoding just before displaying data to avoid mistakes.
- Use Libraries: Frameworks like React or libraries like he (JavaScript) handle encoding safely.
- Test Outputs: Submit <script>alert(‘test’)</script> and verify it displays as text, not a pop-up.
Code Example (JavaScript/HTML for Beginners):
<!DOCTYPE html>
<html>
<body>
<input type="text" id="userInput">
<button onclick="displayInput()">Show</button>
<div id="output"></div>
<script src="https://cdnjs.cloudflare.com/ajax/libs/he/1.2.0/he.min.js"></script>
<script>
function displayInput() {
// Get user input
let input = document.getElementById('userInput').value;
// Encode to prevent scripts
let safeInput = he.encode(input);
// Display safely
document.getElementById('output').innerText = safeInput;
}
</script>
</body>
</html>
Explanation for Beginners: The he.encode() function (from the he library) turns <script> into <script>, so it shows as text, not code. Never use innerHTML for user input—it’s risky! innerText is safer because it doesn’t interpret HTML. Test by typing <script>alert(‘hack’)</script>—it should display as text.
- Content Security Policy (CSP)
CSP restricts which scripts can run on your site, blocking unauthorized ones even if an attacker injects them.
Step-by-Step Instructions:
- Define Trusted Sources: Decide which domains can load scripts (e.g., your own site, self).
- Create a Policy: Write a CSP rule, starting strict (e.g., only allow scripts from your domain).
- Add to Your Site: Include the policy in your server’s HTTP headers or an HTML <meta> tag.
- Test for Violations: Use your browser’s developer tools (F12, Console tab) to spot blocked scripts.
- Monitor and Refine: Log violations to improve your policy over time.
Code Example (HTML for Beginners):
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Security-Policy" content="script-src 'self'; report-uri /csp-report">
</head>
<body>
<script src="my-safe-script.js"></script>
<!-- This won't run if it's from another domain -->
<script src="https://evil.com/malicious.js"></script>
</body>
</html>
Explanation for Beginners: The script-src ‘self’ rule means only scripts from your website (same domain) can run. If someone injects a script from evil.com, the browser blocks it. The report-uri part lets you collect reports if something gets blocked. Add this <meta> tag in your HTML’s <head> section. Check your browser’s Console for errors to confirm it’s working.
- Secure Coding Practices
Writing code with security in mind reduces XSS risks from the start.
Step-by-Step Instructions:
- Learn XSS Risks: Read guides like OWASP’s XSS Prevention Cheat Sheet (owasp.org).
- Avoid Dangerous Functions: Don’t use eval(), document.write(), or innerHTML unless absolutely necessary.
- Use Safe Frameworks: Frameworks like React or Vue.js escape outputs automatically.
- Limit Permissions: Only give scripts the access they need (e.g., don’t let a form script access cookies).
- Review Code: Check your code for mistakes before deploying.
Code Example (JavaScript for Beginners):
// Bad: Using innerHTML (risky)
let userComment = "<p>Hello</p>";
document.getElementById("comment").innerHTML = userComment; // Can run scripts if malicious
// Good: Using textContent (safe)
let safeComment = "<p>Hello</p>";
document.getElementById("comment").textContent = safeComment; // Shows as text
Explanation for Beginners: innerHTML treats input as HTML, so <script> could run. textContent treats it as plain text, preventing scripts. Always use textContent or innerText for user data. If you’re using React, it escapes outputs by default, so {userComment} in a component is safe.
- Regular Security Audits
Audits catch XSS vulnerabilities before attackers do, keeping your app secure over time.
Step-by-Step Instructions:
- Schedule Reviews: Plan to check your code every few months.
- Use Tools: Run free scanners like OWASP ZAP (download from zaproxy.org).
- Update Software: Keep your server, frameworks, and libraries up to date.
- Check Advisories: Look at sites like cve.mitre.org for new XSS threats.
- Learn from Issues: If you find a bug, fix it and note how to avoid it next time.
Example (No Code, But Tool Setup):
- Download OWASP ZAP.
- Open it, enter your website’s URL (e.g., http://yoursite.com).
- Click “Quick Scan” to test for XSS issues.
- Read the report—it lists problems and suggests fixes.
Explanation for Beginners: Tools like ZAP act like a “security robot” that tries to find weak spots in your site. Updates are like patching holes in a fence—do them regularly. If ZAP flags an XSS issue, it might say, “Form input not encoded.” Go back to your code and add encoding (like in step 2).
- HTTP-Only Cookies
Setting cookies as HTTP-only prevents scripts from accessing them, protecting sensitive data like session tokens.
Step-by-Step Instructions:
- Identify Cookies: Find where your app sets cookies (e.g., for logins).
- Add HTTP-Only Flag: Include HttpOnly when creating cookies.
- Use Secure Flag Too: Add Secure to ensure cookies only work over HTTPS.
- Test Access: Try accessing the cookie with JavaScript—it should fail.
- Verify in Browser: Check your browser’s developer tools to confirm the flags.
Code Example (PHP for Beginners):
<?php
// Set a session cookie with HttpOnly and Secure
setcookie('user_session', 'abc123', [
'expires' => time() + 3600, // 1 hour
'path' => '/',
'secure' => true, // Only over HTTPS
'httponly' => true // Blocks JavaScript access
]);
?>
<script>
// Test: This should return undefined
console.log(document.cookie); // Won't show 'user_session'
</script>
Explanation for Beginners: Cookies store data like your login ID. HttpOnly means JavaScript can’t touch them, so even if an XSS script runs, it can’t steal your session. Secure ensures the cookie only works on secure sites (HTTPS). Check in your browser’s developer tools (F12, Application > Cookies) to see the flags.
- Use XSS-Protecting Libraries
Libraries like DOMPurify sanitize HTML inputs, ensuring only safe content is allowed.
Step-by-Step Instructions:
- Pick a Library: Use DOMPurify for JavaScript (available via CDN or npm).
- Include in Project: Add the library to your HTML or install it.
- Sanitize Inputs: Run user HTML through the library before displaying it.
- Set Rules: Configure what tags or attributes to allow (e.g., <p> but not <script>).
- Test Thoroughly: Submit malicious HTML and verify it’s cleaned.
Code Example (JavaScript for Beginners):
<!DOCTYPE html>
<html>
<body>
<textarea id="userHTML"></textarea>
<button onclick="showHTML()">Display</button>
<div id="safeOutput"></div>
<script src="https://cdnjs.cloudflare.com/ajax/libs/dompurify/3.1.6/purify.min.js"></script>
<script>
function showHTML() {
let input = document.getElementById('userHTML').value;
// Sanitize with DOMPurify
let cleanHTML = DOMPurify.sanitize(input, { ALLOWED_TAGS: ['p', 'b'] });
// Display safely
document.getElementById('safeOutput').innerHTML = cleanHTML;
}
</script>
</body>
</html>
Explanation for Beginners: DOMPurify removes dangerous HTML, like <script> tags, but keeps safe ones you allow (here, <p> and <b>). Type <p>Hello</p><script>alert(‘hack’)</script> into the textarea—it’ll show “Hello” but ignore the script. Add the library via the <script src> link, and you’re ready to go.
Conclusion
Preventing XSS attacks requires vigilance and a multi-layered approach. By implementing input validation, output encoding, CSP, secure coding, regular audits, HTTP-only cookies, and sanitization libraries, you create a robust defense against malicious scripts. Each measure targets a different angle—blocking bad inputs, neutralizing outputs, restricting scripts, and maintaining vigilance. For beginners, start small: add validation to one form, encode one output, and test with tools like ZAP. Security is a journey—keep learning, testing, and updating to stay ahead of attackers. Your users’ trust depends on it.